- Every C compiler in String handling functions in C supports a large number of String Handling Library functions.
- When we use string library functions in our program then we have to specify #include <string.h> in our program.
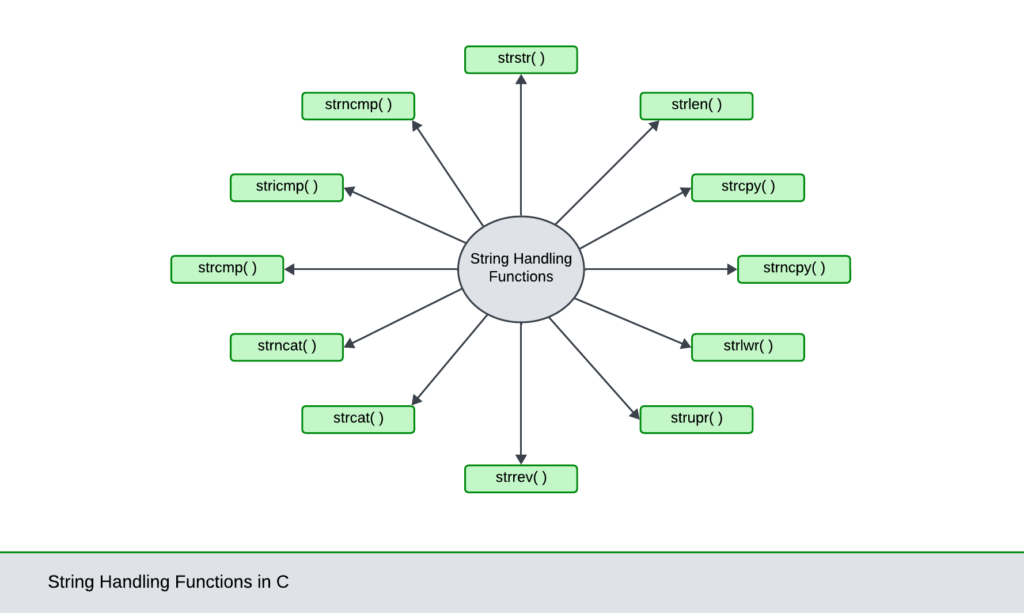
- The most commonly used String handling Functions in C are
strlen(): This function is used to find the length of the string, which means that it counts the number of characters in a string.
The syntax is strlen(string);
Ex: strlen(“Murthy”);
strcpy(): This function is used to copy the contents of one string to another.
The syntax is strcpy(string1, string2); where string2 is the source string and string1 is the target string.
Ex: char str1[20]=”Malya”, str2[20]=”Vijay”;
strcpy(str1, str2);
vijay
strncpy(): This function is used to copy the number of characters from string 2 to string 1.
The syntax is strncpy(string1,string2, n);
Ex: char str1[20], str2[20] = “sachin”;
strncpy(str1,str2, 4);
str1=sach
str2=sachin
strlwr(): This function is used to convert the characters of a string from upper case to lower case.
The syntax is strlwr(string);
strlwr(“DHONI”); dhoni
strupr(): This function is used to convert the characters of a string from lowercase to uppercase.
The syntax is strupr(string);
strupr(“dhoni”); DHONI
strrev(): This function is used to display the characters of a string in reverse order.
The syntax is strrev(string);
strrev(“Murthy”); yhtruM
strcat(): This function is used to concatenate the two strings. Concatenation means adding the two strings.
The syntax is strcat(string1, string2);
where string2 is concatenated with string 1 and the result is stored in string1.
strcat(“Virat”, “Kohli”);
strncat(): This function is used to concatenate the specified number of characters of string 2 to string 1 and the result is stored in string 1.
The syntax is strncat(string1, string2, n);
strncat(“Sachin”, “Tendulkar”, 3);
strcmp(): This String handling Function in C used to compare the two strings which means that it checks whether the two strings are identical or not.
This function is used to compare the strings based upon the ASCII values of each character in the string.
The strcmp() function returns a value of zero or non-zero.
If it returns zero value the strings are identical. Otherwise, the strings are not identical.
The syntax is
strcmp(string1, string2);
strcmp(“MURTHY”, “MURTHY”);
strcmp(“abc”, ”abc”); 🡪0
strcmp(“JNTUH”, “JNTUK”); 🡪-3
strcmp(“JNTUK”, “JNTUH”); 🡪 3
stricmp(): This function is similar to strcmp() function. Except that it does not discriminate between the upper case letters and lower case letters.
The syntax is stricmp(string1, string2);
stricmp(“murthy”, “murthy”); 🡪0
strncmp(): This function is similar to strcmp() function. But it compares the two strings up to a specified number of characters.
The syntax is strncmp(“MURTHY”, “Murthy”, 3); 🡪 -32
strncmp(“rama”, “ramu”, 3); 🡪0
strstr(): This function is used to find the position of string 2 in string 1. If string 2 is not present in string 1 it returns a value -1.
The syntax is strstr(string1, string2);
strstr(“ramaraju”, “raju”); 🡪4strstr(“sachin”, “chin”); 🡪2
FAQs on String handling Functions in C
What is the difference between
strcpy()
andstrncpy()
in C?Both copy strings, but
strncpy()
takes a third argument specifying the maximum number of characters to copy. This can help prevent buffer overflow errors.How can I find the length of a string in C?
Use the
strlen()
function from the<string.h>
header. It returns the number of characters in the string (excluding the null terminator).What are the different ways to compare strings in C?
strcmp()
performs a case-sensitive comparison.strncmp()
compares up to a specified number of characters.stricmp()
(orstrcasecmp()
) performs a case-insensitive comparison.How do I concatenate (combine) strings in C?
Use the
strcat()
function to append one string to the end of another. Be sure the destination string has enough space to hold the combined result.What is the safest way to get user input for a string in C?
Use
fgets()
instead ofscanf("%s")
.fgets()
allows you to specify the maximum size of the input buffer, preventing buffer overflow attacks.