- A loop statements in C is defined as a block of statements which are repeatedly executed for a certain number of times until the condition is satisfied.
- The different types of looping statements in C language are while loop, do while loop, and for loop.
- Loops are either event-controlled or counter-controlled.
- In an event-controlled loop, an event occurs whenever the expression is evaluated.
- Ex: while, do while.
- A counter-controlled loop is used to specify how many times the loop will be executed.
- Ex: for loop
When we declare a loop we have to consider three things.
- Loop variable: The Variable which is used in the loop as well as to control the loop is called a loop variable.
- Loop Variable initialization: Once the loop variable has been declared it has to be assigned some value either with an initial value or final value.
- Loop variable incrementation/decrementation: It is a numerical value added or subtracted to the loop variable in each iteration of the loop. Each time the updated value of the loop variable is compared with the expression.
Loop Statements in C are:
- for loop
- while loop
- do while loop
Table of Contents
for loop in c:
- For loop is another repetitive control structure. for loop is a pretest loop.
- For loop is used as a counter-controlled loop.
for loop syntax in c
for(expression 1; expression 2; expression 3;)
{
Statement 1;
Statement 2;
….
Statement n;
}
- The for statement contains 3 expressions. The first expression contains the initialization statement, the second expression contains the text condition, and the third expression contains the updated value of the loop variable.
- The initialized counter sets a loop to an initial value and it is executed only once.
- The text condition is a relational expression that determines the number of times the loop will be executed.
- The loop variable is incremented or decremented depending on the initial value.
- If there is only one statement in the for loop there is no need to specify open brace and closed brace.
- If there are multiple statements in the for loop then we have to specify open and closed brace.
Flowchart:
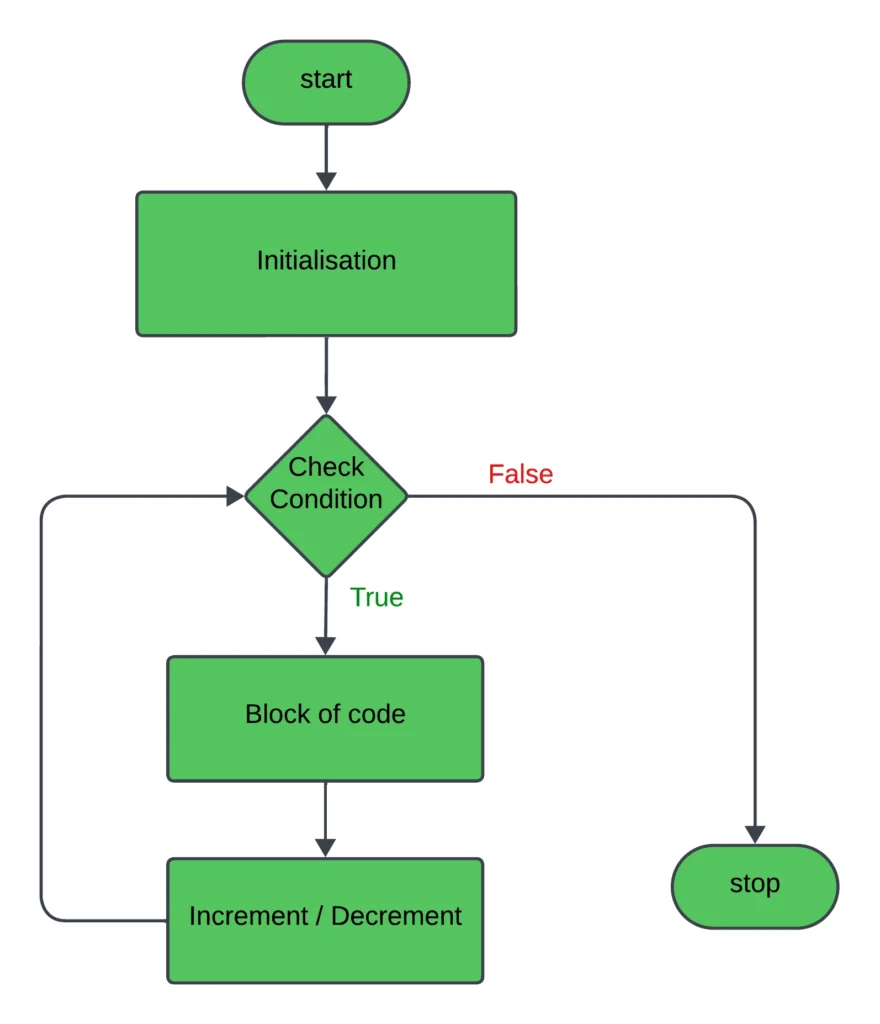
for loop example in c
Write a Programme to print the odd numbers from 1 to n using for loop.
#include <stdio.h> int main() { int n, i; printf("Enter the value of n: "); scanf("%d", &n); printf("Odd numbers from 1 to %d are: ", n); for (i = 1; i <= n; i += 2) { // Start at 1, increment by 2 in each iteration printf("%d ", i); // Directly print the odd numbers without the need for the if condition } printf("\n"); return 0; }
Output:
Enter the value of n: 12
Odd numbers from 1 to 12 are: 1 3 5 7 9 11
While loop in C:
- While loop is known as entry controlled loop or pre-test loop.
- While loop is a repetitive controlled structure to execute the statements within the loop until the condition becomes false.
While loop syntax in C:
While (expression)
{
Statement 1;
Statement 2;
…..
Statement n;
}
while loop flowchart in C
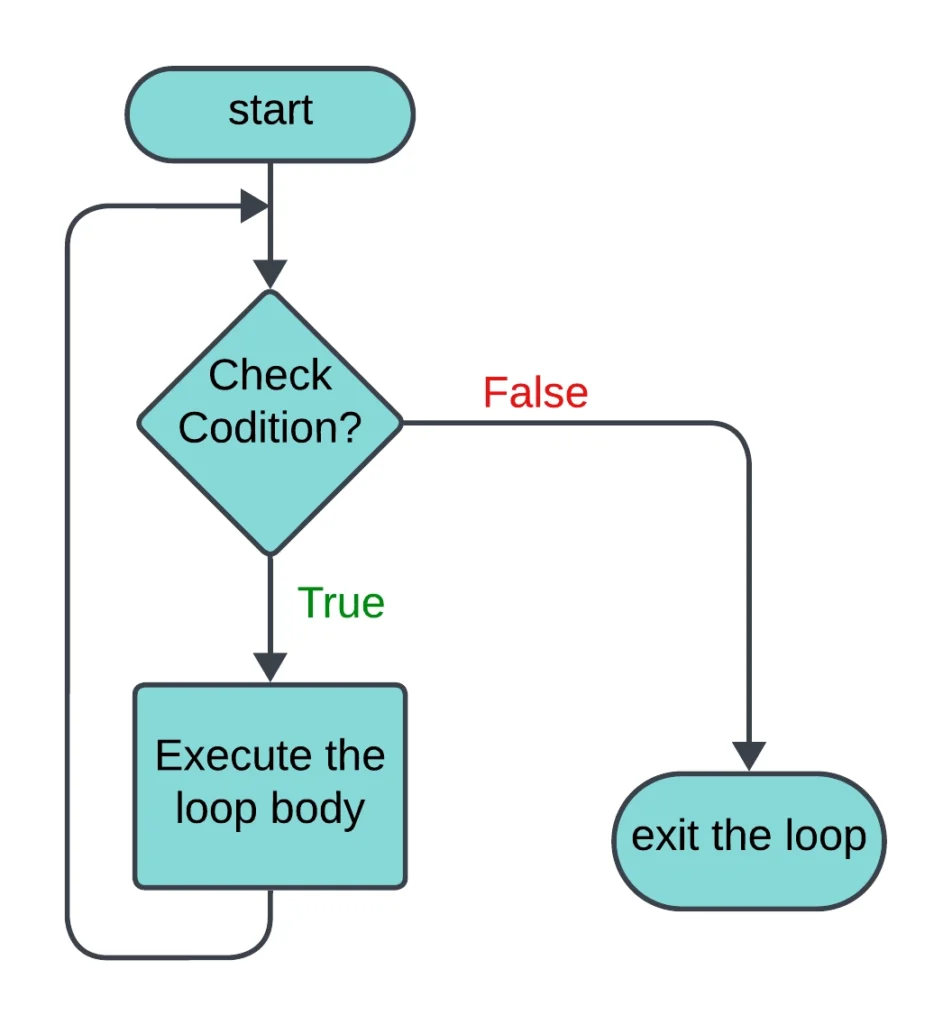
While loop example in C
Write a program to print the first 20 natural numbers.
#include <stdio.h> int main() { // Variable Declaration and Initialization int counter = 1; // Initialize a counter to keep track of the current number // Header for the output printf("The first 20 natural numbers are:\n"); // While Loop to Print the Numbers while (counter <= 20) { printf("%d ", counter); // Print the current number counter++; // Increment the counter for the next iteration } printf("\n"); return 0; // Indicate successful program execution }
Output:
The first 20 natural numbers are:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
do while loop in C
- Do while loop is another repetitive control structure which executes the body of the loop at least once irrespective of the condition.
- After executing the body of the loop it evaluates the expression. If it generates true value again it enters into the loop and executes the body of the loop.
- Once again the expression is evaluated. If it results in a false value it comes out of the loop.
do while loop syntax in C
do
{
int 1;
int 2;
int 3;
….
int n;
while (expression);
do while flowchart in C
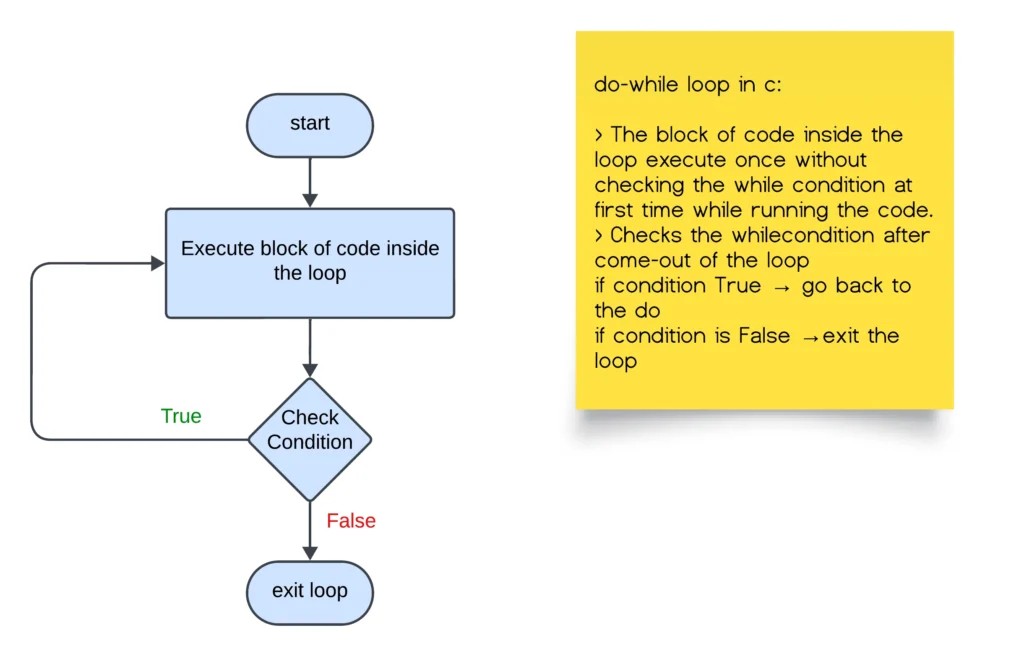
do while loop in c programming example
#include <stdio.h> int main() { // Variable Declaration and Initialization int counter = 1; // Initialize a counter to keep track of the current number // Header for the output printf("The first 20 natural numbers are:\n"); // Do-While Loop to Print the Numbers do { printf("%d ", counter); // Print the current number counter++; // Increment the counter for the next iteration } while (counter <= 20); // Continue the loop while counter is less than or equal to 20 printf("\n"); return 0; // Indicate successful program execution }
Output:
The first 20 natural numbers are:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20
loop control statements in c
- Loop control statements in C programming are used to change execution from its normal sequence.
- In C programming, loop control statements serve the purpose of altering the natural flow of execution.
Break Statement :
- In programming, the break statement allows for premature loop or switch termination, transferring execution to the subsequent statement.
Continue Statement
- The continue statement bypasses the rest of the body and resets its condition before reiterating.
Goto Statement
- The goto statement allows for direct transfer of control to a specific labeled statement.