- An Array is a collection of similar data items that are stored under a common name.
- An Array in C programming can be declared in the declarative part of the program.
Table of Contents
Array Syntax in C
data type array name [size];
Ex: int a[5];
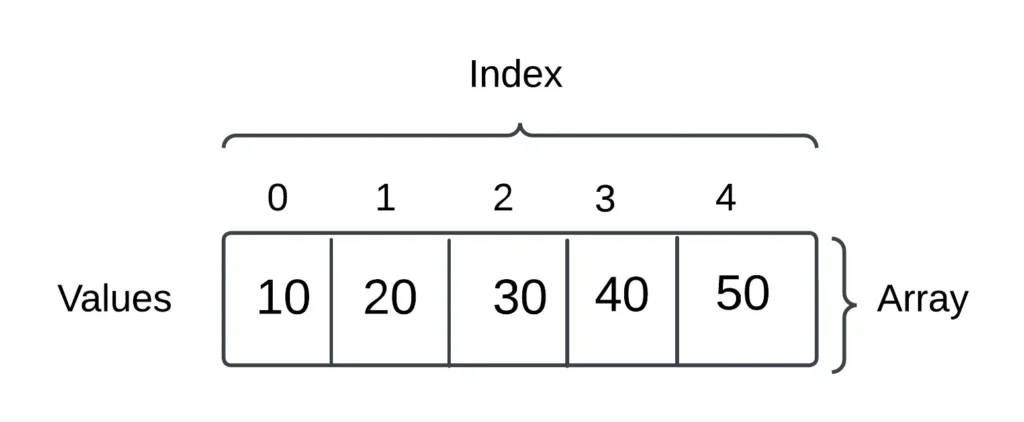
- [0]🡪subscript.
- 10, 20, 30, 40, and 50 are values of an array.
- 0, 1, 2, 3, 4, 5 🡪index number
- an🡪array name
- [0] – 10 🡪 rows in the table indicate the location of an array.
- Each location of an array indicated by a number is called an Index number.
- The index number which is enclosed within the square brackets is called subscript.
- The subscript of an array always starts from 0.
- The subscript of an array does not contain negative values. The values in an array may be of integer data type, real data type (float), or character data type.
- The subscript of an array is always associated with its array name.
Types of Array in C programming
There are two types of arrays based on the number of dimensions it has. They are as follows
1.One Dimensional Arrays(1D Array)
2. Multidimensional Arrays
1.One Dimensional Arrays(1D Array)
- An array which has only one subscript is called a 1-D array. In a 1-D array, the values are stored in different rows but only in one column.
- The 1-D array can be declared as global or local.
- Initializing the array: Like other variables, we can also initialize a 1-D array at the time of declaration.
- Ex: int a[5] ={10,20,30,40,50};
- The elements in the array are stored sequentially in separate memory locations.
Syntax of 1D Array in C
array_name[size];
Example of 1D Array in C
#include <stdio.h> int main() { // Declare an array of integers with 5 elements int arr[5]; // Initialize the elements of the array arr[0] = 10; arr[1] = 20; arr[2] = 30; arr[3] = 40; arr[4] = 50; // Access and print elements of the array printf("Elements of the array:\n"); for (int i = 0; i < 5; i++) { printf("arr[%d] = %d\n", i, arr[i]); } return 0; }
Output
Elements of the array:
arr[0] = 10
arr[1] = 20
arr[2] = 30
arr[3] = 40
arr[4] = 50
2. Multidimensional Arrays
- Multi-dimensional Arrays in C are those arrays that have more than one dimension.
- Some of the popular multidimensional arrays are 2D arrays and 3D arrays.
- We can declare arrays with more dimensions than 3d arrays but they are avoided as they get very complex and occupy a large amount of space.
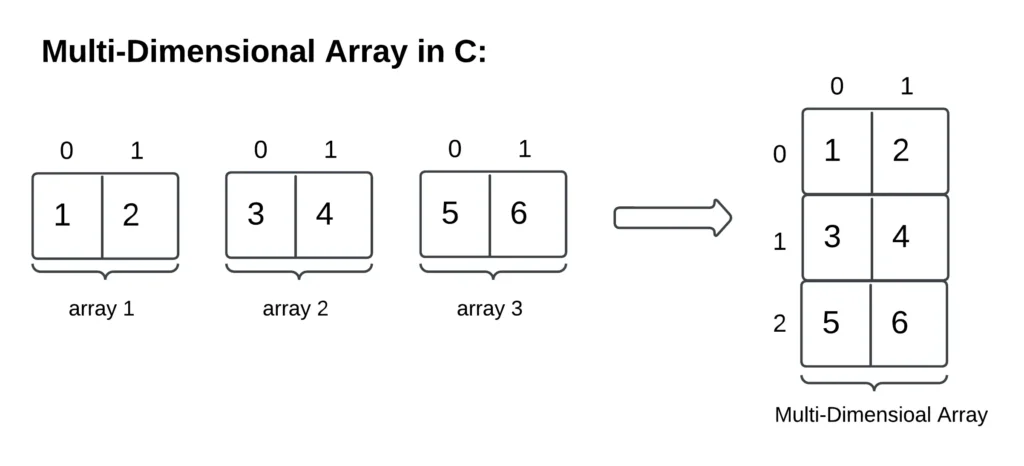
Two Dimensional Array in C(2D array)
- A Two-Dimensional array or 2D array in C is an array that has exactly two dimensions.
- They can be visualized in the form of rows and columns organized in a two-dimensional plane
Syntax of 2D Array in C
Data type array name [size1][size2];
Example of 2D Array in C
#include <stdio.h> int main() { // Declare and initialize a 2D array of integers with 2 rows and 3 columns int arr[2][3] = { {1, 2, 3}, // First row {4, 5, 6} // Second row }; // Access and print elements of the 2D array printf("Elements of the 2D array:\n"); for (int i = 0; i < 2; i++) { for (int j = 0; j < 3; j++) { printf("arr[%d][%d] = %d\n", i, j, arr[i][j]); } } return 0; }
Output
Elements of the 2D array:
arr[0][0] = 1
arr[0][1] = 2
arr[0][2] = 3
arr[1][0] = 4
arr[1][1] = 5
arr[1][2] = 6
Three Dimensional Array in C(3D Array)
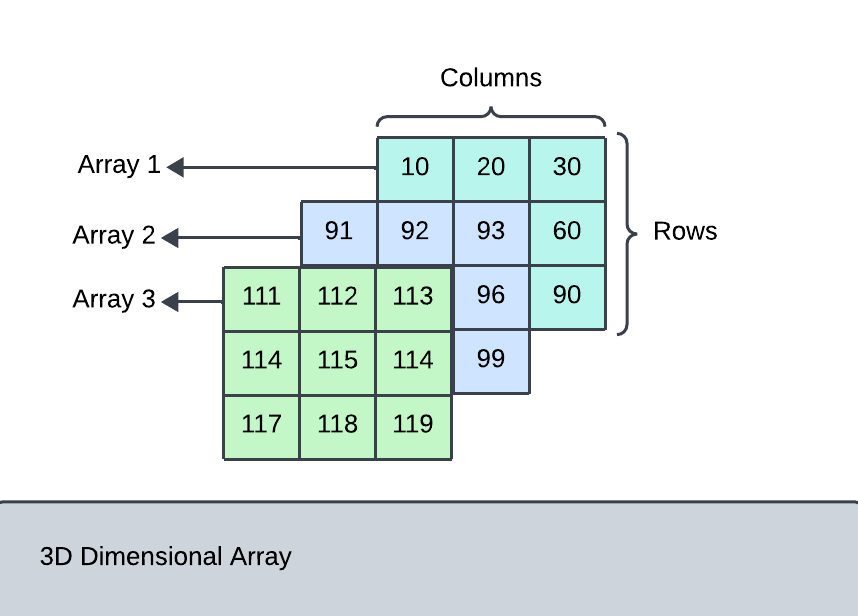
- A 3D array has exactly three dimensions.
- It can be visualized as a collection of 2D arrays stacked on top of each other to create the third dimension.
Syntax of 3D Array in C
Data type array name [size1][size2][size3];
Example of 3D Array in C
#include <stdio.h> int main() { // Declare and initialize a 3D array int arr[2][3][4] = { { // 1st "depth" layer {1, 2, 3, 4}, {5, 6, 7, 8}, {9, 10, 11, 12} }, { // 2nd "depth" layer {13, 14, 15, 16}, {17, 18, 19, 20}, {21, 22, 23, 24} } }; // Access and print elements printf("Element at arr[0][1][2]: %d\n", arr[0][1][2]); // Output: 7 printf("Element at arr[1][2][3]: %d\n", arr[1][2][3]); // Output: 24 // Iterate through all elements and print printf("\nAll elements:\n"); for (int i = 0; i < 2; i++) { for (int j = 0; j < 3; j++) { for (int k = 0; k < 4; k++) { printf("%d ", arr[i][j][k]); } printf("\n"); } printf("\n"); } return 0; }
Output
Element at arr[0][1][2]: 7
Element at arr[1][2][3]: 24
All elements:
1 2 3 4
5 6 7 8
9 10 11 12
13 14 15 16
17 18 19 20
21 22 23 24
Advantages of Array in C programming
Fast Access: Look up any element instantly by its index.
Easy Iteration: Loop through elements effortlessly.
Memory Efficient: Stores elements compactly.
Versatility: A building block for many other data structures.
Performance: Well-suited for sorting and searching.
Disadvantages of Array in C programming
Inflexible Size: Difficult to resize once created.
Slow Insertions/Deletions: Shifting elements is costly for large arrays.
Type Restrictions: Limited to hold elements of the same data type.
FAQs of Array in C
What is an array in programming?
An array is a fundamental data structure used to store a collection of items of the same data type in a contiguous block of memory.
It’s like a numbered list, where each item has a unique index (starting from 0).How do I access elements in an array?
You access array elements using their index.
For example, in the arraynumbers = [10, 25, 5]
, you can get the first element withnumbers[0]
(result: 10).What are the advantages of using arrays?
Arrays offer fast access to elements based on their index, make it easy to store multiple related items, and are the building blocks for many other data structures.
What are the limitations of arrays?
Arrays have a fixed size, meaning you can’t easily add or remove elements once the array is created. Also, they typically store items of only one data type.
How do I find the most frequent element in an array?
You can use techniques like:
Hash Map/Dictionary: Count the occurrences of each element and keep track of the maximum count.
Sorting: Sort the array and look for the longest consecutive run of the same element.